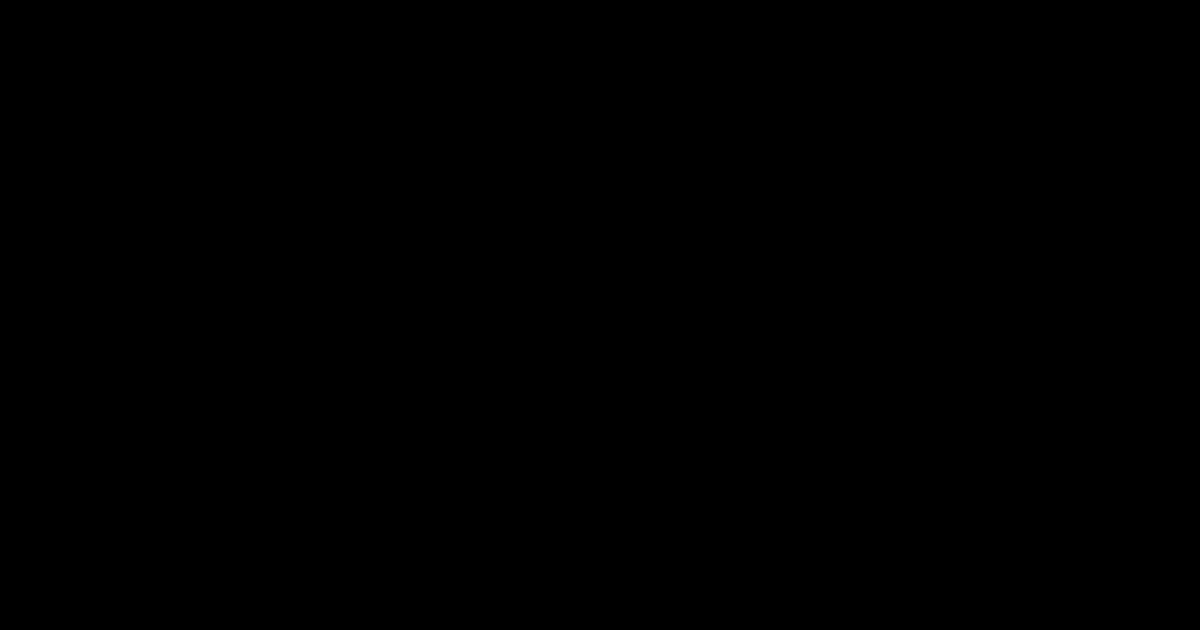
Understanding Spring Security Authorization
Before we dive into the troubleshooting process, let’s take a step back and understand how Spring Security authorization works.
Spring Security’s authorization feature is built around the concept of Authorities
and Roles
. In a nutshell, authorities represent specific permissions within an application, while roles are collections of authorities that define a user’s responsibility.
To authorize an action, Spring Security checks if the user has the required authority or role to perform that action. This check is typically done using the @Secured
annotation at the method or controller level.
Common Scenarios Where Authorization Fails
Now that we have a solid understanding of Spring Security authorization, let’s explore some common scenarios where things can go wrong:
-
Missing or Incorrect Configuration
One of the most common reasons for authorization failure is incorrect or missing configuration. Make sure you have the following components in place:
SecurityContextHolder
: This is the central component that stores the current user’s authentication and authorization information.AuthenticationManager
: This is responsible for authenticating users and retrieving their authorities.AccessDecisionManager
: This component makes the final decision on whether a user has access to a particular resource.
Ensure that these components are properly configured and wired together.
-
Incorrect Authority or Role Definition
Another common pitfall is defining authorities or roles incorrectly. Double-check that your authorities and roles are correctly defined and assigned to users.
@RestController public class MyController { @GetMapping("/admin-only") @Secured("ROLE_ADMIN") public String adminOnly() { return "Hello, admin!"; } }
In this example, the
@Secured
annotation specifies that only users with theROLE_ADMIN
role can access the/admin-only
endpoint. -
Ambiguous Authority or Role Definitions
If you have multiple authorities or roles with similar names, it can lead to ambiguity and authorization failures.
Authority/Role Description ROLE_ADMIN Administrator role ROLE_ADMINISTRATOR alternate administrator role In this example, having two similar roles (
ROLE_ADMIN
andROLE_ADMINISTRATOR
) can cause confusion and lead to authorization issues. Avoid using similar names and ensure that each authority or role has a unique definition.
Troubleshooting Techniques
Now that we’ve covered some common scenarios where authorization might fail, let’s discuss some techniques to troubleshoot and fix these issues:
Enable Debug Logging
One of the most effective ways to diagnose authorization issues is by enabling debug logging for Spring Security. Add the following configuration to your application.properties
file:
logging.level.org.springframework.security=DEBUG
This will provide you with detailed logs that can help you identify the root cause of the authorization failure.
Use the Spring Security Debugger
The Spring Security Debugger is a powerful tool that allows you to visualize the entire authorization process. Add the following dependency to your pom.xml
file (if you’re using Maven):
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
<version>2.3.4.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework.security</groupId>
<artifactId>spring-security-debug</artifactId>
<version>5.3.4.RELEASE</version>
</dependency>
Once you’ve added the dependency, you can access the debugger by visiting /security-debug
in your application.
Verify User Roles and Authorities
Another essential step in troubleshooting authorization issues is to verify that the user has the correct roles and authorities.
@Controller
public class MyController {
@GetMapping("/user-roles")
public String getUserRoles() {
Authentication authentication = SecurityContextHolder.getContext().getAuthentication();
Collection<? extends GrantedAuthority> authorities = authentication.getAuthorities();
// Verify the user's roles and authorities
return "User roles: " + authorities.stream().map(GrantedAuthority::getAuthority).collect(Collectors.joining(", "));
}
}
In this example, we’re retrieving the user’s authorities using the SecurityContextHolder
and verifying that they have the correct roles.
Best Practices for Spring Security Authorization
To avoid common pitfalls and ensure that your Spring Security authorization works as intended, follow these best practices:
Keep Your Configuration Simple and Consistent
Avoid complex configurations that can lead to errors and inconsistencies. Keep your authority and role definitions simple and easy to understand.
Use Meaningful Authority and Role Names
Use meaningful names for your authorities and roles to avoid confusion and ambiguity.
Test Your Authorization Thoroughly
Thoroughly test your authorization configuration to ensure that it works as expected.
Monitor and Debug Your Application
Monitor your application’s logs and debug output to identify and fix authorization issues quickly.
Conclusion
Spring Security authorization can be a powerful tool for securing your application, but it can also be a source of frustration when things don’t work as expected. By following the troubleshooting techniques and best practices outlined in this article, you’ll be well-equipped to identify and fix common authorization issues.
Remember, Spring Security authorization is all about configuring and wiring the right components together. With a solid understanding of authorities, roles, and access decision managers, you’ll be able to create a robust and secure application that meets your requirements.
So, go ahead and take the reins! Spring Security authorization is no longer a daunting task. Happy coding!
Frequently Asked Question
Stuck with Spring Security authorization? Don’t worry, we’ve got you covered! Here are some frequently asked questions and answers about Spring Security authorization not working:
Why is my Spring Security authorization not working after configuring it correctly?
This might be due to the fact that you haven’t enabled Spring Security’s debug logging. Try adding the following configuration to your application.properties file: `debug=true` or `logging.level.org.springframework.security=DEBUG`. This will help you identify the issue by providing more detailed logs.
I’ve implemented custom UserDetailsService, but Spring Security authorization is still not working. What could be the issue?
Make sure you’ve overridden the `loadUserByUsername` method correctly in your custom `UserDetailsService` implementation. Also, ensure that the `UserDetails` object returned by this method contains the correct authorities (roles) for the user. You can debug this by checking the `UserDetails` object in your custom `UserDetailsService` implementation.
I’ve configured Spring Security with OAuth2, but authorization is not working for some users. What could be the issue?
Check if the OAuth2 token is being correctly stored and retrieved for the affected users. You can do this by enabling OAuth2 debug logging or by using a tool like Postman to inspect the OAuth2 token. Also, ensure that the `Principal` object contains the correct authorities (roles) for the user.
I’ve implemented Spring Security with JWT, but authorization is not working. What could be the issue?
Make sure you’ve correctly configured the JWT token validation and extraction. Check if the JWT token is being correctly generated and sent in the requests. Also, ensure that the `JWTTokenValidator` is correctly validating the token and extracting the authorities (roles) for the user.
I’ve configured Spring Security with Method Security, but authorization is not working for some methods. What could be the issue?
Check if you’ve correctly annotated the methods with the `@Secured` or `@PreAuthorize` annotations. Also, ensure that the method security configuration is correctly enabled and configured in your Spring Security configuration file.